A Fairly Fast Fibonacci Function
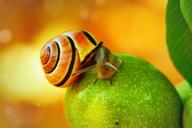
A common example of recursion is the function to calculate the \(n\)-th Fibonacci number:
def naive_fib(n): if n < 2: return n else: return naive_fib(n-1) + naive_fib(n-2) This follows the mathematical definition very closely but it’s performance is terrible: roughly \(\mathcal{O}(2^n)\). This is commonly patched up with dynamic programming. Specifically, either the memoization:
from functools import lru_cache @lru_cache(100) def memoized_fib(n): if n < 2: return n else: return memoized_fib(n-1) + memoized_fib(n-2) or tabulation: